Overview
It's time to grow our app with a new feature: we'll introduce a page that shows a specific track's details. We've gotten some practice querying for a list of tracks—but how do we ask our GraphQL API for one very specific track?
In this lesson, we will:
- Learn about GraphQL arguments
- Write a query that accepts a variable
- Build a page to display data for one track object
A new feature
Our goal is to create a page that requests data for one specific track. To do this, we'll need to put together a query that requests data for a single track.
Let's start with the mockup of the Track page our design team provided us with. Here we'll need to display more information about a track than what's contained in a card on the homepage.
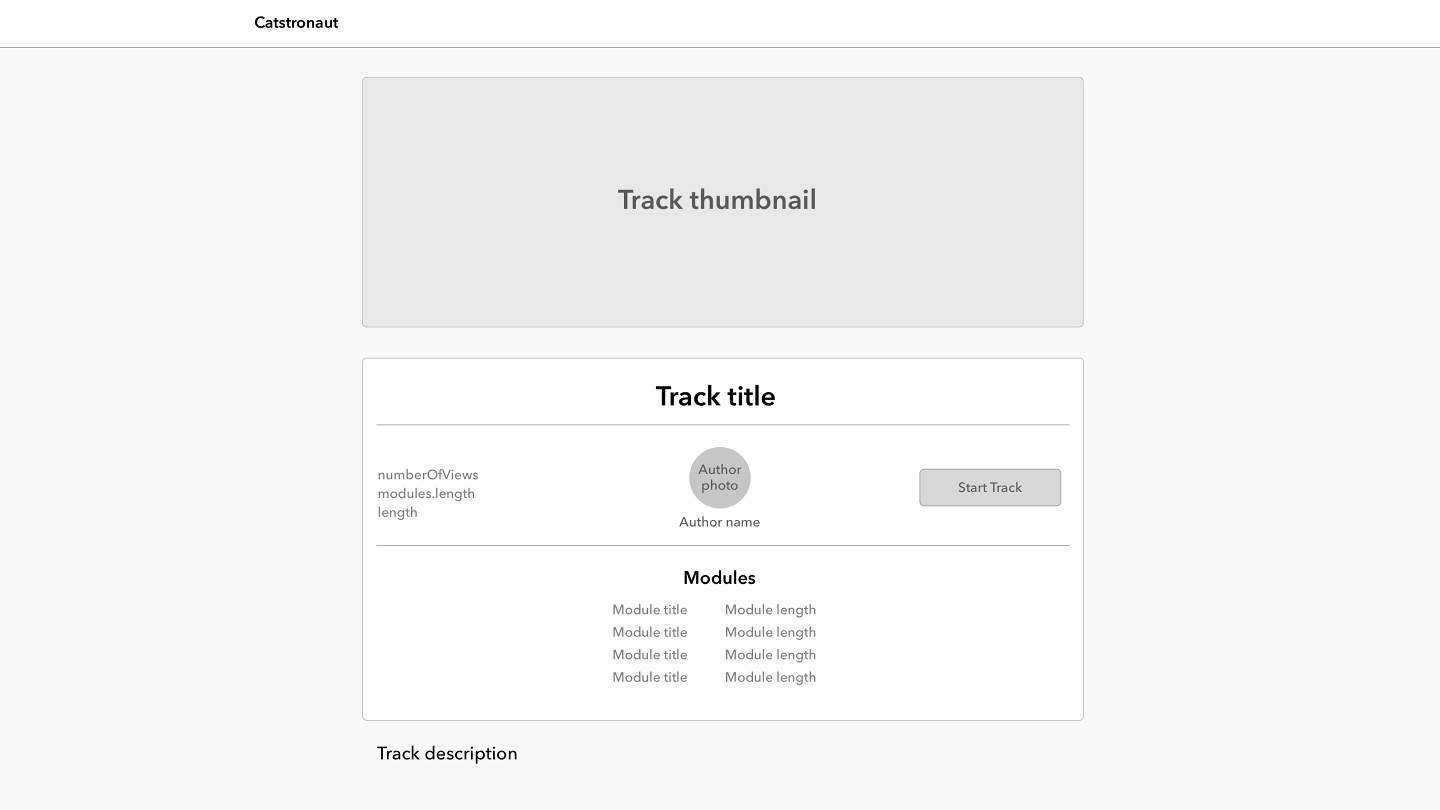
In addition to what we had in our card, a track needs to have:
- a description
- the number of views
- the list of modules included in that track
For each module, we want:
- the title
- the length
A new Query
entrypoint
Let's return to Sandbox, and select the Schema option from the left-hand toolbar.
We've gotten some practice querying the tracksForHome
field, but let's take a look at the two other fields on the Query
type: track
and module
. Each of these remaining fields accepts an argument.
GraphQL arguments
An argument is a value we provide for a particular field in your query. In the case of both track
and module
, the schema defines an id
argument that they each accept. By specifying the id
for a module or a track object, we can query data for a particular object.
track(id: ID!): Track!
On the backend, the GraphQL server can use a field's provided argument(s) to help determine how to populate the data for that field. Arguments can help you retrieve specific objects, filter through a set of objects, or even transform the field's returned value.
The track
field in our schema, which accepts an id
argument, looks like just the field we'll need to use to make our track page dreams come true. It takes an id
, and returns data for a specific Track
!
This means that all we need to do on the frontend is pass a valid value for the id
of a particular track—and the server will take care of returning the data we need!
Let's see how we can build a specific query using arguments in the Explorer.
🛠️ Building our query
Let's jump back to the Explorer and build our query.
Clicking the plus button (⊕) on the track
field, we start to see our query come together in the Operation panel.
First let's rename our query to better explain what it's for, so we'll replace Track
with GetTrack
.
So far, the Operation panel of the Explorer should contain this:
query GetTrack($trackId: ID!) {track(id: $trackId) {}}
You'll notice something new here: a dollar sign ($
) followed by the name trackId
.
đź’° Variables
The $
symbol indicates a variable in GraphQL. The name after the $
symbol is the name of our variable, which we can use throughout the query. After the colon is the variable's type, which must match the type of the argument we'll use it for.
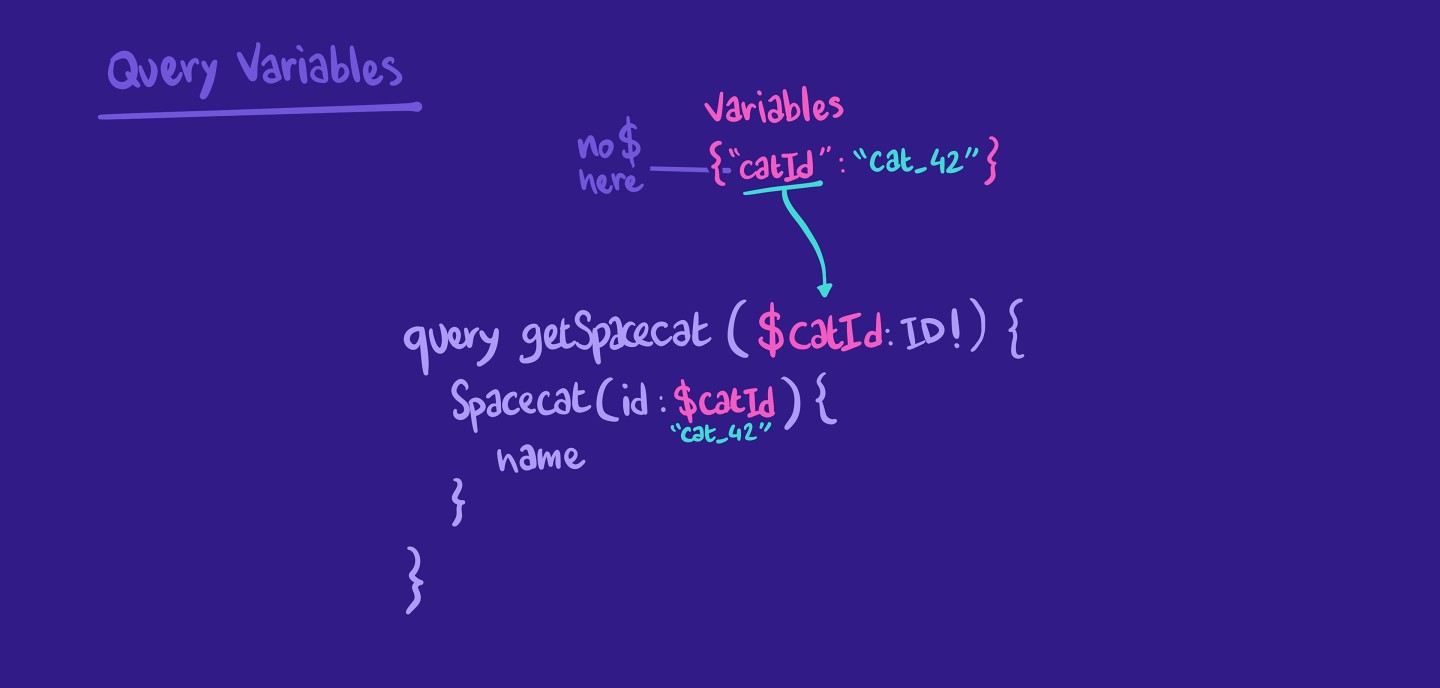
Variables are great—they let us pass argument values dynamically from the client-side so we don't have to hardcode values into our query. We'll use them every time we create a query with arguments.
In our case, we have a variable called trackId
that the Explorer set up for us down in the Variables section. Right now, it's set to null
, but let's replace it with a valid track ID: c_0
.
Add the following to the Variables section in the Explorer:
{ "trackId": "c_0" }
Before we start adding all the fields we need from our initial mockup, let's start small with just returning the id
and the title
.
The Operation panel of the Explorer should now look like this:
query GetTrack($trackId: ID!) {track(id: $trackId) {idtitle}}
When we click on the button to run the query, we see the data we're expecting: the first track's title "Cat-stronomy, an introduction".
Awesome, let's add the rest of our fields by clicking the dropdown by the Fields subheading. When we click Select all fields recursively from the dropdown, we'll see all of our fields and subfields have been added to the query.
The full query should look like this:
query GetTrack($trackId: ID!) {track(id: $trackId) {idtitleauthor {idnamephoto}thumbnaillengthmodulesCountdescriptionnumberOfViewsmodules {idtitlelengthcontentvideoUrl}}}
Let's click on the Run Query button again… it looks like we get the complete track, but it's a bit tricky to read as a JSON object. The Explorer has a nice option to format the response as a table.
And now we can clearly see the track that we need, along with all the details for each module! Nice!
Practice
Drag items from this box to the blanks above
$
hardcoded
@
graph
null
schema
!
arguments
resolvers
name
Build a query called GetMission
. This query uses a variable called isScheduled
of type nullable Boolean
. It retrieves a mission
using the scheduled
argument set to the isScheduled
variable. It retrieves the mission's id
and codename
.
Key takeaways
- A GraphQL argument is a value we provide for a particular field in a query.
- On the backend, the GraphQL server can use arguments to help determine how to populate data for a field.
- We use the
$
symbol to denote a variable in GraphQL. - With variables, we can pass argument values dynamically from the client-side.
Up next
Our query's set, but we still need our frontend pieces! Let's build the track page in the next lesson.
Share your questions and comments about this lesson
This course is currently in
You'll need a GitHub account to post below. Don't have one? Post in our Odyssey forum instead.