Overview
So now we have a plan for our subgraphs! To keep our focus on the Federation-specific concepts, the FlyBy starter repo already comes with base server code. Let's see what's been built so far.
In this lesson, we will:
- Explore what's already been built for the
locations
andreviews
servers - Use the
@apollo/subgraph
package to convert thelocations
andreviews
servers from normal GraphQL servers into subgraphs.
Exploring the starter code
Let's start by checking out what's been built for our subgraphs so far.
The locations
subgraph
We'll start with the locations
subgraph. Here's an architecture diagram showing the files in the subgraph-locations
directory and how they're connected:
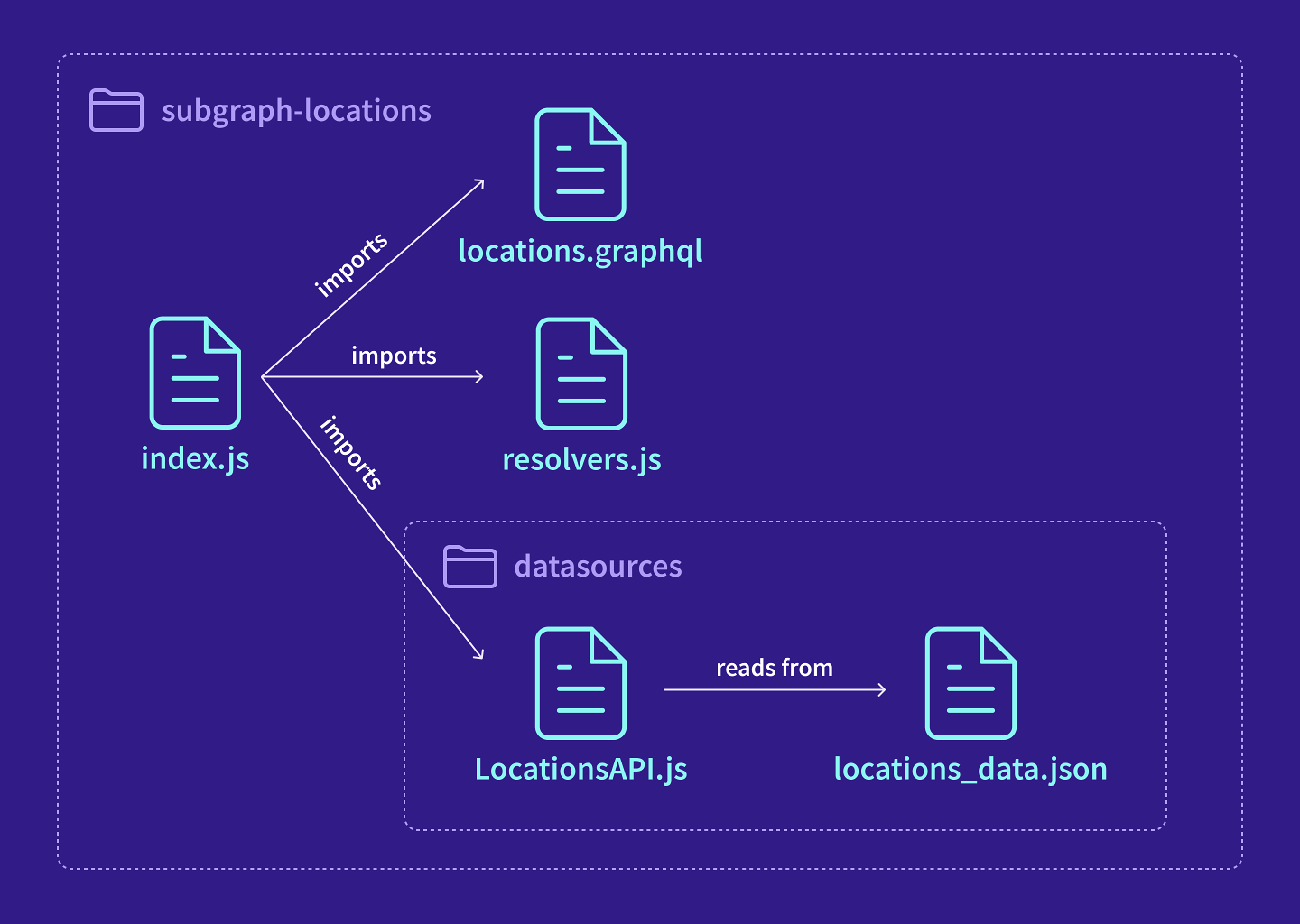
index.js
: Creates anApolloServer
instance that runs on port4001
. So far, this is just a normal GraphQL server, not a subgraph.locations.graphql
: A schema that defines the types and fields owned by thelocations
subgraph.resolvers.js
: Defines resolver functions for the fields in thelocations
schema.datasources/LocationsApi.js
: Retrieves location data from thelocations_data.json
file.- Note: In a real-world application, this data source would talk to a REST API or a database, but we're in tutorial-land so we'll stick with the hard-coded fake data.
datasources/locations_data.json
: A JSON object with hard-coded location data.
Take a moment to look over these files and familiarize yourself with their contents.
Let's update our schema agreement checklist with the fields that have been added to our locations
subgraph so far:
The reviews
subgraph
Next up, the reviews
subgraph. The files in the subgraph-reviews
directory have a similar structure to our locations
subgraph.
Let's skip ahead and once again update our schema agreement checklist. We'll mark off the fields that have been added to our reviews
subgraph so far.
Remember, we'll leave the three fields (Location.reviewsForLocation
, Location.overallRating
and Review.location
) alone for now, but we'll get to them later on in the course!
✏️ Starting up the subgraph servers
Let's get these subgraph servers up and running. First, the locations
subgraph.
From the command line, navigate to the
subgraph-locations
directory:cd subgraph-locationsStart the
locations
server by running the following command:npm startYou should see a success message like the one below:
🚀 Subgraph locations running at http://localhost:4001/Before we hop on over to check out our subgraph, let's rename this terminal window to
subgraph-locations
, to make it easier to come back to our running server later.In a web browser, go to http://localhost:4001 to open your server in Apollo Sandbox. Let's test that the
locations
server is working correctly by running the following query:query GetAllLocations {locations {idnamedescriptionphoto}}When we run this query, we can see that the locations server sends back our data correctly, perfect!
Now let's start up the reviews
subgraph, which will follow similar steps!
Open a new terminal window, and navigate to the
subgraph-reviews
directory:cd subgraph-reviewsStart the
reviews
server by running the following command:npm startYou should see a success message like the one below:
🚀 Subgraph reviews running at http://localhost:4002/
Let's also rename this terminal window to be subgraph-reviews
to make it easy to find again later.
Open another browser tab to
http://localhost:4002
and query your server using Sandbox.We'll test that the
reviews
server is working correctly by running the following query:query GetLatestReviews {latestReviews {idcommentrating}}And we get back data. Huzzah!
Converting to subgraph servers
So far, our locations
and reviews
servers are just regular ol' GraphQL servers, but we're about to convert them into Official Subgraph Servers!
This requires two steps:
- Adding a Federation 2 definition to our subgraph schema files
- Updating our
ApolloServer
instances
✏️ Federation 2 definition
Let's tackle the locations
subgraph first.
Open up the locations.graphql
file, and paste in this Federation 2 definition at the top of the file:
extend schema@link(url: "https://specs.apollo.dev/federation/v2.7",import: ["@key"])
This lets us opt into the latest features of Apollo Federation. It also lets us import the various directives we'd like to use within our schema file (like the @key
directive, shown above). We'll cover the @key
directive later, so don't worry too much about this syntax now.
If your terminal is showing errors at this point, don't worry. Those will go away after we finish up the next step.
✏️ Updating our ApolloServer
instance
The next step is to update our ApolloServer
implementation.
Let's start with the locations
server:
In a new terminal window, navigate to the
subgraph-locations
directory.Run the following command to install the
@apollo/subgraph
package. (This will add@apollo/subgraph
to thepackage.json
file andnode_modules
directory.)npm install @apollo/subgraphOpen the
subgraph-locations/index.js
file in a code editor. Import thebuildSubgraphSchema
function from@apollo/subgraph
.subgraph-locations/index.jsconst { ApolloServer } = require("@apollo/server");const { startStandaloneServer } = require("@apollo/server/standalone");const { buildSubgraphSchema } = require("@apollo/subgraph");Down below, where we initialized the
ApolloServer
, we're now going to pass the existingtypeDefs
andresolvers
properties as an object into thebuildSubgraphSchema
function.Then we'll use the result to set a new
ApolloServer
configuration property calledschema
.subgraph-locations/index.jsconst server = new ApolloServer({schema: buildSubgraphSchema({ typeDefs, resolvers }),});
What's going on with the buildSubgraphSchema
function?
The buildSubgraphSchema
function takes an object containing typeDefs
and resolvers
and returns a federation-ready subgraph schema. This schema includes a number of federation directives and types that enable our subgraph to take full advantage of the power of federation. More on that in a bit!
When we save our changes to the server file, the locally running
locations
server should restart automatically. Now let's check that everything is working correctly! Let's go back to our browser window with Apollo Sandbox athttp://localhost:4001
.Under the
Query
root type, we should see a new field,_service
. This is one of the federation-specific fields thatbuildSubgraphSchema
adds to the subgraph. The router uses this field to access the SDL string for your subgraph schema. We won't use this field directly, but seeing it appear in the Explorer tells us that our subgraph is running correctly.
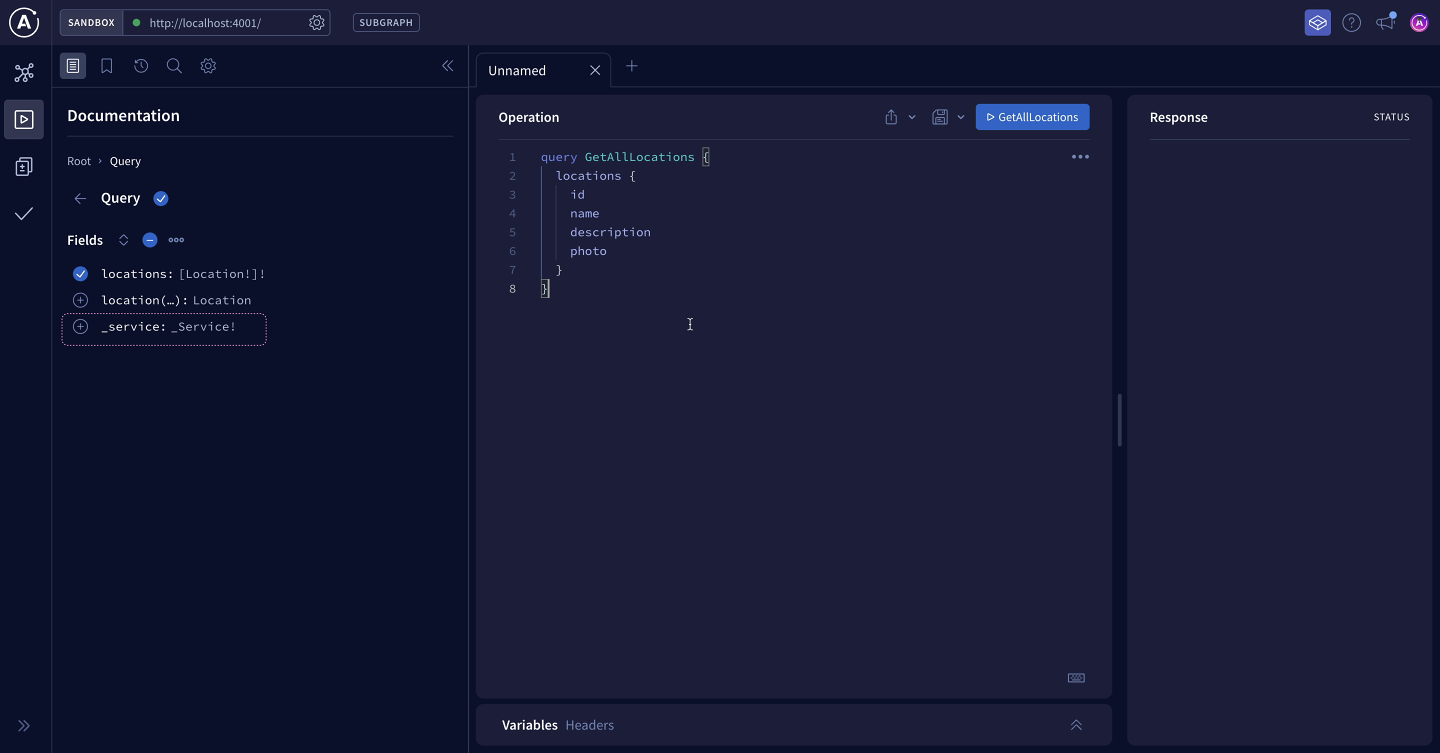
Now that we've set up the locations
subgraph, it's time to repeat the process for the reviews
subgraph! Just follow the same flow as before. You got this.
Hint: If you get stuck, you can always check the final
directory for a hint.
Practice
ApolloServer
instance a subgraph, we install a package called ApolloServer
configuration object using the Drag items from this box to the blanks above
fields
resolvers
@apollo/federation
subgraph schema
@apollo/subgraph
buildSubgraphSchema
typeDefs
@apollo/server
router
buildSupergraph
dataSources
schema
Key takeaways
- Adding a Federation 2 definition to the top of our schema file lets us opt in to the latest features available in Apollo Federation 2.
- To make an
ApolloServer
instance a federation-ready subgraph, use thebuildSubgraphSchema
function from the@apollo/subgraph
package.
Up next
We've got our subgraphs up and running! But that's only the first piece of our supergraph architecture.
In the next lesson, we'll take a closer look at how we can use managed federation to pull all the pieces of our supergraph together.
Share your questions and comments about this lesson
Your feedback helps us improve! If you're stuck or confused, let us know and we'll help you out. All comments are public and must follow the Apollo Code of Conduct. Note that comments that have been resolved or addressed may be removed.
You'll need a GitHub account to post below. Don't have one? Post in our Odyssey forum instead.